I love the Newsletter Glue plugin for WordPress. Before I found it, I had tinkered for a long time with different imperfect solutions to what seemed like a simple need: quickly and easily send people an engaging, visually appealing email when I publish a new article on my website.
I’ve tried manually sending out Mailchimp campaigns after publishing (too slow and cumbersome to customize per post), using messages sent directly from my WordPress host server (too prone to being flagged as spam), automatically generating Mailchimp campaigns from an RSS feed (limited scheduling options and prone to errors), using WordPress.com new post notifications via Jetpack (not very customizable), developing my own plugin to send on demand via MailChimp’s API (very functional but not easily re-usable across sites), and others.
Alas, nothing felt quite like what I wanted.
I’d revisit the challenge now and then, especially when I’d see everybody over there loving on Substack like “oh isn’t amazing how you can send out a readable email newsletter from a website, what an innovation” and found myself rolling my eyes especially hard. “SURELY WE SHOULD BE ABLE TO DO THAT WITH WORDPRESS!” I’d exclaim. And then my family would look at me with tilted heads before going back to whatever they were doing.
I was about to start putting some serious time into turning my own proof of concept Mailchimp API plugin into something reusable and shareable, when I did one last round of research into existing options. And that’s when I found Newsletter Glue. The sky opened up. Light shone down. I did a dance. At least that’s what it felt like. (I see that Justin Tadlock at WP Tavern has had a similar experience.)
So, yes, ahem, where was I? Oh, right: Newsletter Glue is an elegant solution to a real need in the world of WordPress publishing. Go check it out if you haven’t already. I bought a 5-site license and sent Lesley Sim, one of the plugin’s co-creators, a note of appreciation.
The rest of this blog post is about a few additional Newsletter Glue customizations I set up. While some of this is possible via the Newsletter Glue UI, I did it via a small custom plugin that I could re-use across all of my WordPress sites without additional configuration.
I basically wanted to be able to generate and iterate on a simple, sparse, Om Malik-inspired post notification email using the same general setup on every site. Logo, date, title, featured image, and post body.
As an example, for this blog post, here’s the delightful Newsletter Glue-powered end result as seen in my macOS email client:
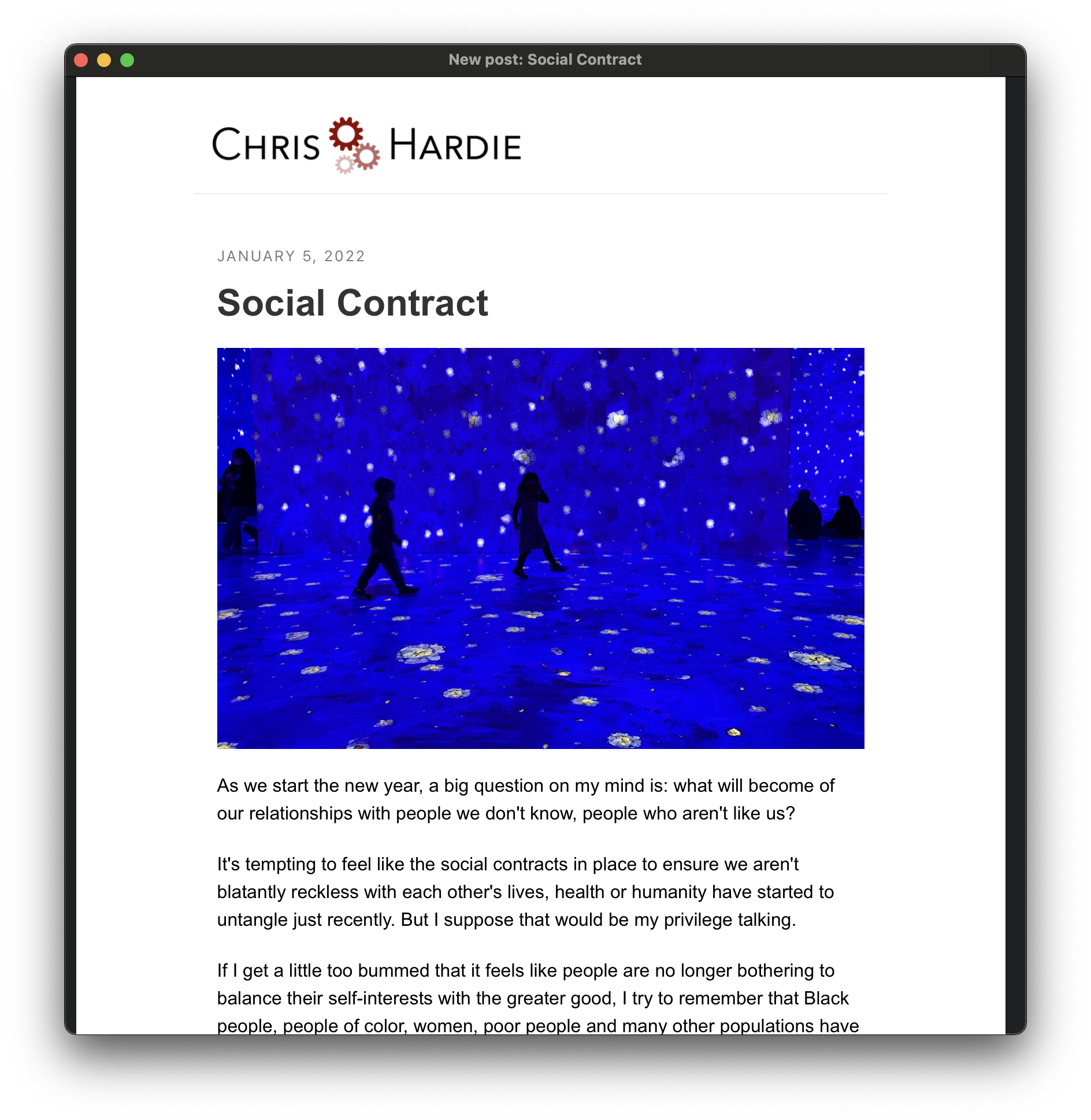
At the bottom of the email message, I just wanted a standard footer that would be ready to go with the right text and links based on the site where the post was published:
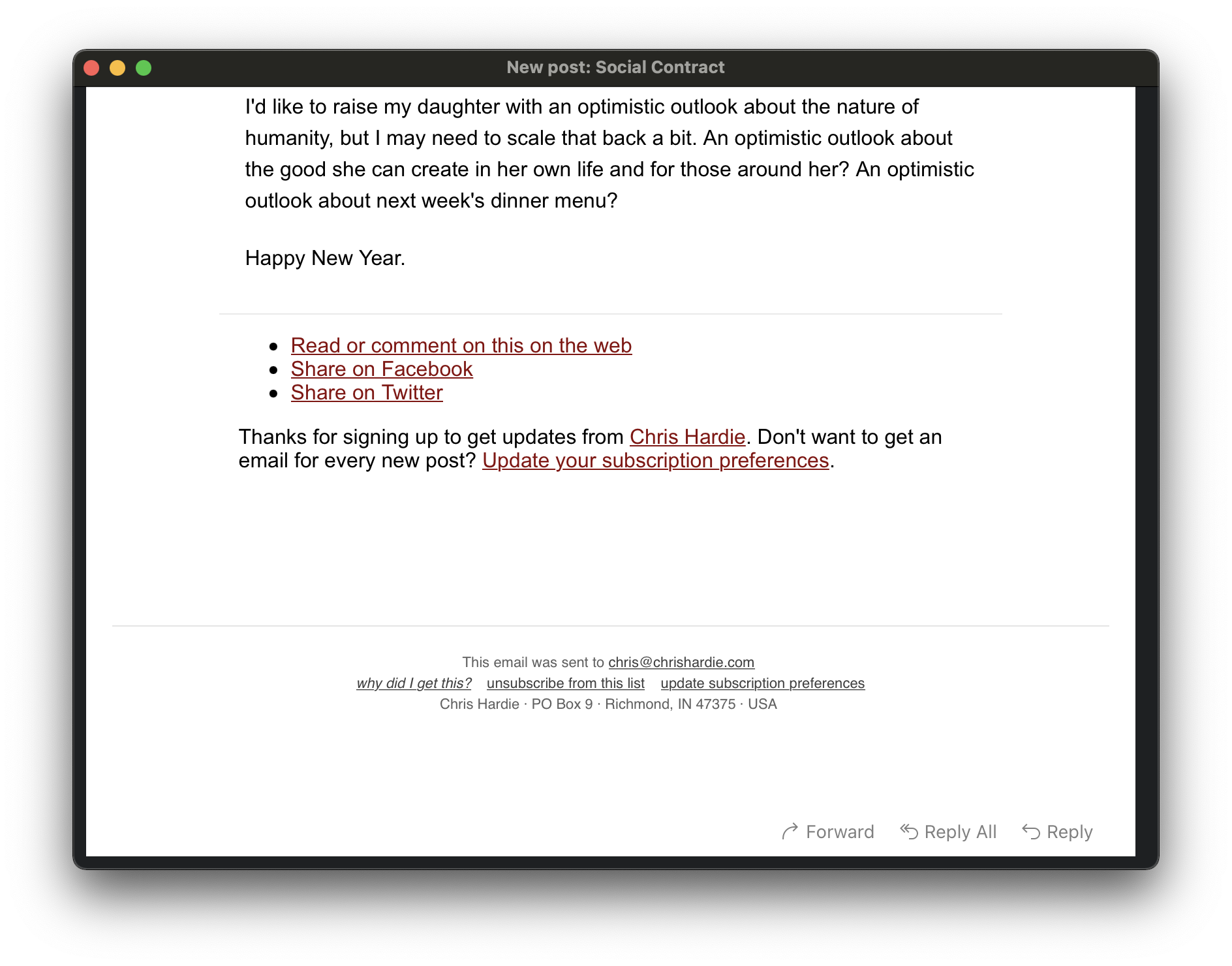
Here’s the basic plugin code that achieves this, using available Newsletter Glue filters:
<?php
class JCH_Newsletter_Glue_Customization_Email_Content {
protected $logo_url = null;
protected $logo_height = null;
protected $logo_width = null;
protected $twitter_handle = null;
protected $permalink = null;
protected $twitter_share_url = null;
protected $facebook_share_url = null;
protected $site_config = array(
'chrishardie.com' => array(
'logo_url' => 'https://chrishardie.com/wp-content/uploads/2018/05/chris-logo-unpadded-480.png',
'logo_width' => 480,
'logo_height' => 90,
'twitter_handle' => '@ChrisHardie',
),
'tech.chrishardie.com' => array(
'logo_url' => 'https://tech.chrishardie.com/wp-content/uploads/2020/06/chris-tech-logo-300x80.png',
'logo_width' => 300,
'logo_height' => 80,
'twitter_handle' => '@ChrisHardie',
),
);
public function __construct() {
$site_domain = wp_parse_url( site_url(), PHP_URL_HOST );
$this->set_vars( $site_domain );
add_filter( 'newsletterglue_email_content_header', array( $this, 'generate_header' ), 10, 3 );
add_filter( 'newsletterglue_email_content_footer', array( $this, 'generate_footer' ), 10, 3 );
}
public function set_vars( $site_domain = null ): void {
if ( ! empty( $site_domain ) && ! empty( $this->site_config[ $site_domain ] ) ) {
$this->logo_url = $this->site_config[ $site_domain ]['logo_url'];
$this->logo_width = $this->site_config[ $site_domain ]['logo_width'];
$this->logo_height = $this->site_config[ $site_domain ]['logo_height'];
$this->twitter_handle = $this->site_config[ $site_domain ]['twitter_handle'];
} else {
$this->set_vars( 'chrishardie.com' );
}
}
public function generate_header( $content, $app, $post ) {
ob_start();
include plugin_dir_path( __DIR__ ) . 'partials/header.php';
$header_content = ob_get_clean();
$content .= $header_content;
return $content;
}
public function generate_footer( $content, $app, $post ) {
$this->set_social_share_links( $post );
ob_start();
include plugin_dir_path( __DIR__ ) . 'partials/footer.php';
$footer_content = ob_get_clean();
$content .= $footer_content;
return $content;
}
public function set_social_share_links( $post ) {
$this->permalink = get_the_permalink( $post );
$this->twitter_share_url = add_query_arg(
array(
'url' => get_the_permalink( $post ),
'text' => get_the_title( $post ) . ' by ' . $this->twitter_handle,
),
'https://twitter.com/intent/tweet'
);
$this->facebook_share_url = add_query_arg(
array(
'u' => get_the_permalink( $post ),
),
'https://www.facebook.com/sharer/sharer.php'
);
}
}
new JCH_Newsletter_Glue_Customization_Email_Content();
In the mentioned header and footer files, it was important to maintain the table-based approach to layout that Newsletter Glue employs in order to be maximally compatible with various mail delivery tools and email reading clients.
Header:
<?php
?>
<table style="...">
<tbody>
<tr>
<td style="...">
<a href="<?php echo esc_url( site_url() ); ?>"><img src="<?php echo esc_url( $this->logo_url ); ?>"
height="<?php echo esc_attr( $this->logo_height ); ?>"
width="<?php echo esc_attr( $this->logo_width ); ?>"
alt="<?php echo esc_html( get_bloginfo( 'name' ) ); ?> Logo"
class="jch-ng-logo"
style="..."
/></a>
</td>
</tr>
</tbody>
</table>
<p style="..."> </p>
<table align="left" border="0" cellpadding="0" cellspacing="0" style="...">
<tbody>
<tr>
<td valign="top" style='...'>
<div>
<span class="metadata post-date" style='...'>
<?php echo get_the_date( 'F j, Y', $post ); ?>
</span>
</div>
</td>
</tr>
</tbody>
</table>
And footer:
<?php
?>
<table align="left" border="0" cellpadding="0" cellspacing="0" style="...">
<tbody>
<tr>
<td valign="top" style='...'>
<ul>
<li><a href="<?php echo esc_url( $this->permalink ); ?>">Read or comment on this on the web</a></li>
<li><a href="<?php echo esc_url( $this->facebook_share_url ); ?>">Share on Facebook</a></li>
<li><a href="<?php echo esc_url( $this->twitter_share_url ); ?>">Share on Twitter</a></li>
</ul>
<p>
Thanks for signing up to get updates from <a href="<?php echo esc_url( site_url() ); ?>"><?php echo esc_html( get_bloginfo( 'name' ) ); ?></a>.
Don't want to get an email for every new post? <a href="*|UPDATE_PROFILE|*">Update your subscription preferences</a>.
</p>
</td>
</tr>
</tbody>
</table>
I’m so happy with the result. I can write a blog post, preview it in my browser, preview the email version in my browser or with a test email, and then publish AND send out the email (in my case, still using Mailchimp but without logging in to their site) right from within the WordPress post editor.
Best of all, since starting to use this approach last year, I’ve noticed that my new posts get a lot more engagement from the folks who receive these email notifications than they did before. Keeping that great writing-publishing-feedback-conversation cycle going is, at least for me, what blogging with WordPress is all about.
Nice! Om’s post emails are powered by ConvertKit’s RSS -> email functionality with a custom template.
What are your thoughts on the best way to make Newsletter Glue a paid newsletter subscription like Substack’s paid offering?
Thanks, Chuck. My main experience with paid subscriptions in WP is WooCommerce’s Subscriptions and Memberships plugins. I love their flexibility but I think piecing those together with a NG setup is probably too much to ask a typical site owner to do. I’d love to see a bundled version that makes “pay to sign up for my newsletter” really easy to get going.
There are probably other plugins/services that do this really well and I’m just not familiar with them yet. Any suggestions?