Over on my newspaper publisher blog I recently shared about things we’ve been doing to automate pieces of the newspaper’s print layout process:
One of them that was in progress at the time was a script to fetch articles and story content from our Airtable-managed story database for faster placement on the page, instead of copying and pasting.
That script is now in production and while it still has some rough edges, is also open sourced on GitHub. I thought I’d go into a little more detail here about how it works:
- Layout editor opens a page file they want to do layout on
- Layout editor runs InDesign script
- Script determines what page is being worked on based on the filename
- Script makes a call to a remote API with the page number as a query parameter, to see what stories are available and ready to be placed on that page, and gets them as a JSON data structure
- Using a base story layer that exists in our page template, script creates a new story layer with the headline, subhead, byline, story content, etc. filled in from the JSON data
- Script finds and replaces Markdown syntax in the content with established InDesign styles for bold, italics, body subheaders, bullet points, etc.
- Layout editor drags the new layer(s) into place, adjusts dimensions, and marks the stories as placed
- Rinse and repeat
We’re also working toward adding support for images and captions/cutlines.
This turns what could be a tens or hundreds of clicks process for a given story into just a few clicks. It saves more time on some stories than others, but especially for the ones that involved a lot of applying inline character styles that were being lost or mangled during copy/paste, I think it’s a clear win.
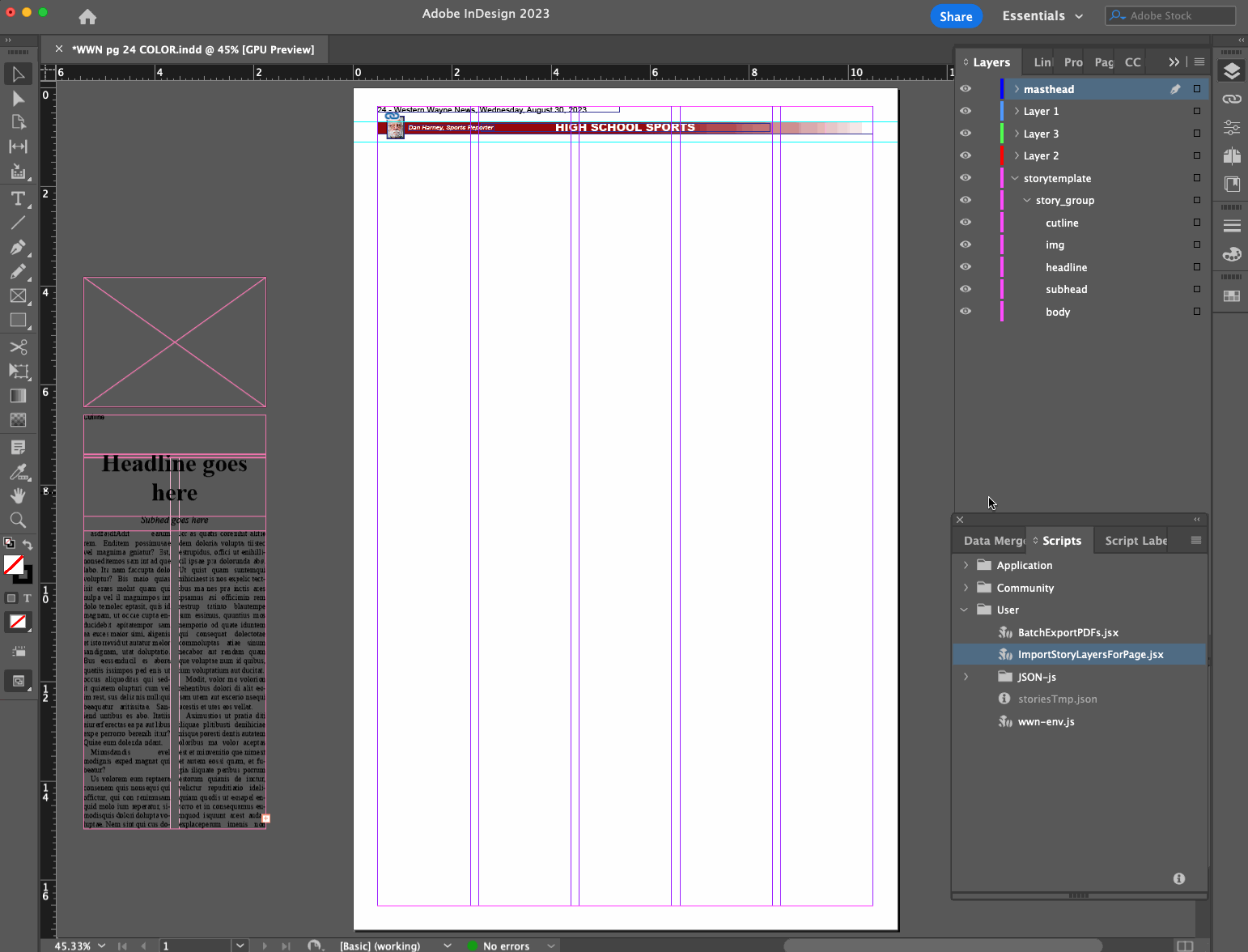
I referred to a “remote API” above because even though our stories are managed in Airtable right now, I chose to introduce an intermediate API for the InDesign script to call for simplicity and so that we weren’t locked in to Airtable’s way of doing things.
In our case, it’s implemented as a single action controller in Laravel, which essentially proxies the query on to the Airtable API and maps out a new, simpler data structure from the result with some content cleanup thrown in along the way:
$airtableResults = $this->getAirtableStories($pageNumber, $nextIssueDate);
$stories = $airtableResults->map(function (array $item) {
$content = $this->processContent($item['fields']['Story Content']);
return [
'id' => $item['id'],
'headline' => $this->extractHeadlineFromContent($content, $item['fields']['Story']),
'subhead' => $this->extractSubtitleFromContent($content),
'byline' => $this->getByline($item),
'body' => $content,
];
});
return response()->json([
'status' => 'success',
'count' => $stories->count(),
'data' => $stories->toArray(),
]);
You can tell that there are some hacky things going on with how we represent headlines, subheads and bylines in the content of our stories, but that’s a blog post for another time.
Here’s what a response might look like:
{
"status": "success",
"count": 2,
"data": [
{
"id": "record_id_1",
"headline": "My first headline",
"subhead": null,
"byline": "From staff reports",
"body": "Lectus viverra cubilia.",
"image_url": "https://placehold.co/600x400",
"cutline": "My image caption 1 here"
},
{
"id": "record_id_2",
"headline": "A second story",
"subhead": "You should really read this",
"byline": "By David Carr",
"body": "Lectus viverra cubilia.",
"image_url": null,
"cutline": null
}]
}
The README at the root of the repository explains further how the API query and response should work and includes some basic installation instructions. (If you use this, I recommend including some light caching so that you don’t over-query the Airtable API, or wherever your stories are stored.)
I realize everyone’s layout and story management tools are different and that the likelihood that our workflow matches up with yours is slim…but just in case this is helpful to anyone else, I wanted to get it out there! Let us know what you think.